パスワード生成時によくある入力条件を満たしたらボタンを押せるようになるというのをVue.jsで実装してみたいと思います。
■仕様
・入力文字は
a.8文字以上
b.英大文字と英小文字を含む
c.数字を含む
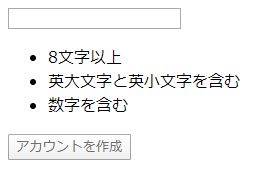
・上記仕様を満たしていることを分かるようにする
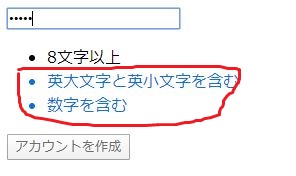
・上記仕様全て満たしたら「アカウント作成」ボタンを押せるようにする
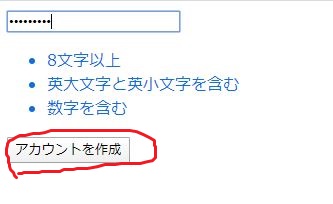
■jQueryとVueをインクルード
<script src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
■本体
<style>
.checked{
color:#0E6FE5;
}
</style>
<div id="app">
<input id="password" type="password" v-model="password">
<ul>
<li v-bind:class="{ checked: isLength }">8文字以上</li>
<li v-bind:class="{ checked: isAlphabet }">英大文字と英小文字を含む</li>
<li v-bind:class="{ checked: isExistNumeric }">数字を含む</li>
</ul>
<input type="submit" name="submitConfirm" class="login_btn submit_btn" v-bind:disabled="isSubmitActive" value="アカウントを作成">
</div>
- 3つの条件は、それぞれ’isLength’,’isAlphabet’,’isExistNumeric’にバインディング
- 「アカウント作成」ボタンも3つの条件をクリアするまで押せないよう’isSubmitActive’にバインディング
■Vue
$(function(){
var app = new Vue({
el: '#app',
data: {
password: '',
},
computed: {
isLength: function() {
if(this.password.length >= 8) return true;
else return false;
},
isAlphabet: function() {
return (this.password.match(/[A-Z]/) && this.password.match(/[a-z]/)) ? true : false;
},
isExistNumeric: function() {
return this.password.match(/[0-9]/) ? true : false;
},
isSubmitActive: function() {
return (this.isLength && this.isAlphabet && this.isExistNumeric) ? false : true;
},
}
})
});
- passwordテキストボックスは、passwordにバインディングされている為、値が変化するたびにcomputedメソッドが実行される
- computed中のメソッドのtrueが返ってきたもの(条件がクリアしたもの)は条件の色が変わる
- 全ての条件クリアで「アカウント作成」ボタンが押下可能になる
Vue.jsを使えばこんなシンプルな実装で可能になる。
■全てのソース
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8" />
<script src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
<body>
<style>
.checked{
color:#0E6FE5;
}
</style>
<div id="app">
<input id="password" type="password" v-model="password">
<ul>
<li v-bind:class="{ checked: isLength }">8文字以上</li>
<li v-bind:class="{ checked: isAlphabet }">英大文字と英小文字を含む</li>
<li v-bind:class="{ checked: isExistNumeric }">数字を含む</li>
</ul>
<input type="submit" name="submitConfirm" class="login_btn submit_btn" v-bind:disabled="isSubmitActive" value="アカウントを作成">
</div>
<script>
$(function(){
var app = new Vue({
el: '#app',
data: {
password: '',
},
computed: {
isLength: function() {
if(this.password.length >= 8) return true;
else return false;
},
isAlphabet: function() {
return (this.password.match(/[A-Z]/) && this.password.match(/[a-z]/)) ? true : false;
},
isExistNumeric: function() {
return this.password.match(/[0-9]/) ? true : false;
},
isSubmitActive: function() {
return (this.isLength && this.isAlphabet && this.isExistNumeric) ? false : true;
},
}
})
});
</script>
</body>
</html>
他にも条件を追加したければcomputedにメソッドを追加すればいいし
文字を入力するたびにパスワード強度が「弱い」->「普通」->「強い」と表示が変化するようなことも難しくない。